Python is known for its simplicity and versatility, making it a great choice for both beginners and experienced developers. One of the lesser-known yet incredibly fun libraries in Python is turtle
. The turtle
module is a standard Python library used to introduce programming to kids and beginners, but it’s also capable of creating some pretty impressive graphics.
In this blog, we’ll explore what Python Turtle is, how to get started, and some cool examples of graphics you can create with it. All source code is available on GitHub.
What is Python Turtle?
Python Turtle is a graphical library that allows you to draw shapes, patterns, and images using code. The idea behind it is simple: you control a “turtle” that moves around the screen, drawing lines as it goes. You can make the turtle move forward, turn, change colors, and much more.
Getting Started with Python Turtle
To start using Turtle, you first need to import the module. You can do this with a simple import statement:
import turtle
Next, you’ll want to create a screen and a turtle:
# Set up the screen
screen = turtle.Screen()
screen.bgcolor("white") # Set the background color
# Create a turtle
my_turtle = turtle.Turtle()
my_turtle.shape("turtle") # You can set the shape to 'turtle', 'arrow', etc.
my_turtle.color("blue") # Set the color of the turtle
With these few lines of code, you’ve set up your environment and are ready to start drawing!
Example 1: Drawing a Simple Square
Let’s start with something simple: a square.
import turtle
# Set up the screen
screen = turtle.Screen()
screen.setup(width=400, height=400)
screen.bgcolor("black") # Set the background color to black
# Create a turtle
my_turtle = turtle.Turtle()
# Draw a square
for _ in range(4):
my_turtle.forward(100) # Move forward by 100 units
my_turtle.right(90) # Turn right by 90 degrees
# Keep the window open until clicked
turtle.done()
This code will draw a simple square. The turtle moves forward by 100 units, turns 90 degrees to the right, and repeats this four times to complete the square.
Here is a sample output:
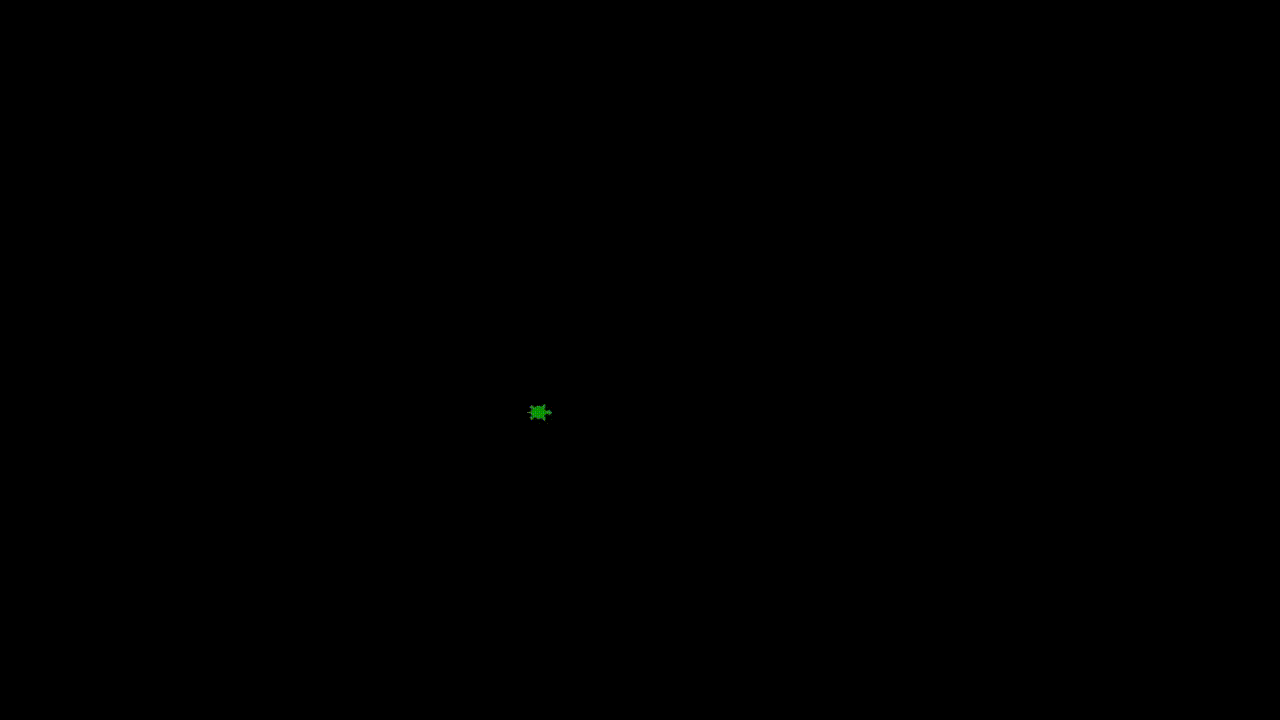
Example 2: Drawing a Star
Now, let’s create something a bit more complex: a star.
import turtle
# Set up the screen
screen = turtle.Screen()
screen.setup(width=400, height=400)
screen.bgcolor("black") # Set the background color to black
# Create a turtle
star_turtle = turtle.Turtle()
star_turtle.color("red")
# Draw a star
for _ in range(5):
star_turtle.forward(150) # Move forward by 150 units
star_turtle.right(144) # Turn right by 144 degrees
# Keep the window open until clicked
turtle.done()
The key to drawing the star is turning the turtle by 144 degrees each time, which creates the star shape after five iterations.
Example 3: Creating a Spiral
Let’s create a cool spiral pattern. This example will combine colors and gradually increase the length of each line segment.
import turtle
# Create a turtle
spiral_turtle = turtle.Turtle()
colors = ["red", "purple", "blue", "green", "orange", "yellow"]
# Draw a spiral
for i in range(100):
spiral_turtle.color(colors[i % 6]) # Cycle through colors
spiral_turtle.forward(i * 5) # Increase the length of each segment
spiral_turtle.right(144) # Turn right by 144 degrees
# Keep the window open until clicked
turtle.done()
This code creates a mesmerizing spiral pattern with colors that change as the spiral grows. The length of each line segment increases with every loop iteration, and the turtle turns by 144 degrees, which results in a star-like spiral.
Example 4: Creating a Mandala Pattern
For something more intricate, let’s create a mandala pattern.
import turtle
# Create a turtle
mandala_turtle = turtle.Turtle()
mandala_turtle.speed(0) # Set the turtle's speed to the fastest
colors = ["red", "blue", "green", "orange", "purple", "yellow"]
# Draw a mandala pattern
for i in range(36):
mandala_turtle.color(colors[i % 6]) # Cycle through colors
mandala_turtle.circle(100) # Draw a circle with a radius of 100
mandala_turtle.right(10) # Turn right by 10 degrees
# Keep the window open until clicked
turtle.done()
This code generates a beautiful mandala by drawing 36 circles, each rotated slightly from the previous one. The result is a colorful and complex pattern that looks like it took much more effort than it actually did!
Conclusion
The Python Turtle module is a fantastic way to create graphics and learn programming at the same time. Whether you’re a beginner looking to understand loops and functions or an experienced coder interested in making generative art, Turtle provides an accessible and enjoyable way to do it.
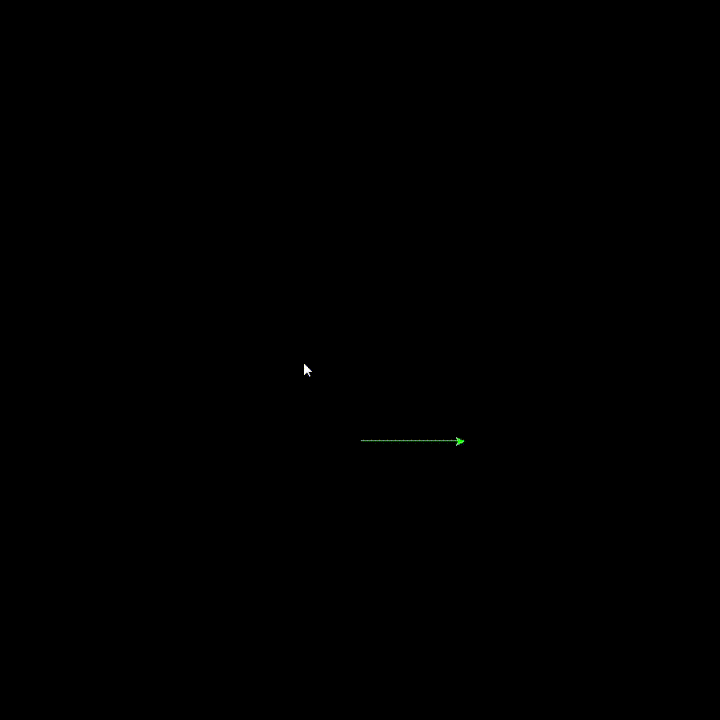
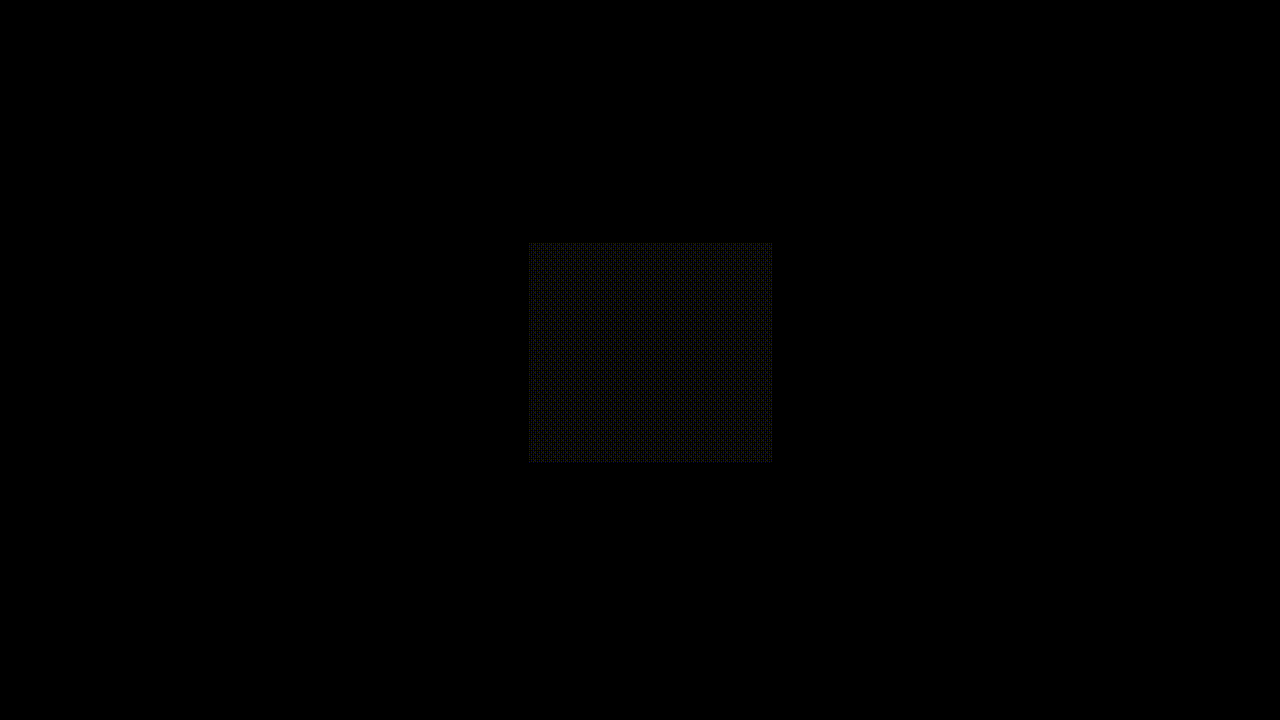
Try experimenting with different shapes, patterns, and colors to see what unique designs you can create. Happy coding!